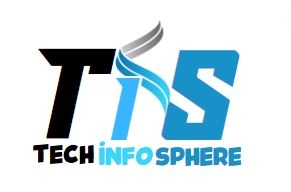
Learn how to create an HTML table with a 100% width and a scrollable <tbody>, keeping the headers fixed for improved user experience.
When working with large data sets in web development, especially when you're displaying them in tables, one common challenge is how to handle scrolling without losing sight of the table headers. You may want the table to have 100% width while allowing the vertical scrolling inside the <tbody>
. In this post, we'll walk you through how to achieve this using HTML and CSS.
Tables with a large number of rows can overwhelm the user interface, making it difficult to scroll and reference the headers. A solution to this is making the <tbody>
scrollable while keeping the headers fixed at the top, improving the user experience by keeping key information in view.
Here’s how you can achieve a table with a 100% width and vertical scrolling inside the <tbody>
.
Let’s start by setting up a basic HTML table structure:
<div class="table-container">
<table class="table-fixed">
<thead>
<tr>
<th>Header 1</th>
<th>Header 2</th>
<th>Header 3</th>
<th>Header 4</th>
</tr>
</thead>
<tbody>
<tr>
<td>Data 1</td>
<td>Data 2</td>
<td>Data 3</td>
<td>Data 4</td>
</tr>
<!-- More rows go here -->
</tbody>
</table>
</div>
<tbody>
Next, let’s use CSS to ensure the table has a fixed width and the <tbody>
becomes scrollable vertically.
.table-container {
max-width: 100%;
height: 400px; /* Adjust based on your needs */
overflow: auto; /* To allow scrolling */
}
.table-fixed {
width: 100%;
border-collapse: collapse;
}
thead th {
position: sticky;
top: 0;
background-color: #f8f8f8;
}
tbody {
display: block;
max-height: 300px; /* Set the max height for scrolling */
overflow-y: scroll; /* Enables vertical scrolling */
}
tbody tr {
display: table;
width: 100%;
table-layout: fixed; /* Ensures the columns align */
}
thead, tbody tr {
display: table;
width: 100%;
table-layout: fixed;
}
Container Styling: The .table-container
class ensures the table takes up 100% of its parent’s width. The height
is defined to allow scrolling when the content overflows vertically.
Table Styling: .table-fixed
ensures the table maintains its width and has no gaps between cells due to the border-collapse
property.
Sticky Header: Using position: sticky;
on the <thead>
ensures the headers stay fixed at the top when the user scrolls through the <tbody>
.
Scrollable <tbody>
: The key part is to set the <tbody>
to display: block;
, giving it a fixed height and setting overflow-y: scroll;
. This allows only the body of the table to scroll while keeping the header in place.
<div class="table-container">
<table class="table-fixed">
<thead>
<tr>
<th>Header 1</th>
<th>Header 2</th>
<th>Header 3</th>
<th>Header 4</th>
</tr>
</thead>
<tbody>
<tr>
<td>Row 1 Data 1</td>
<td>Row 1 Data 2</td>
<td>Row 1 Data 3</td>
<td>Row 1 Data 4</td>
</tr>
<tr>
<td>Row 2 Data 1</td>
<td>Row 2 Data 2</td>
<td>Row 2 Data 3</td>
<td>Row 2 Data 4</td>
</tr>
<!-- More rows... -->
</tbody>
</table>
</div>
Improved Usability: Users can scroll through the data without losing sight of the table headers, ensuring they know what each column represents at all times.
Responsiveness: With width: 100%
applied to the table, it adapts well to different screen sizes, keeping the table easy to read on both small and large displays.
Flexibility: You can control the height of the table and how many rows are visible at a time by simply adjusting the max-height
of the <tbody>
.
This method works well for most use cases but has a couple of potential limitations:
position: sticky
, though most modern browsers do.<thead>
and <tbody>
.If you're dealing with large data sets in a table, implementing a scrollable <tbody>
with a fixed header can dramatically enhance the user experience. By following the steps outlined above, you can create tables that are both functional and user-friendly.
Was this guide helpful? Share your thoughts in the comments, and don’t forget to like and share if you found it useful! Keep following for more tips on web development.